Documentation
How to create a new Block
In this tutorial a creation of a joystick block will be descripted, which access the axis and buttons of a joysting using the external library libSDL.Module
At first you need a module inside of the HART-toolbox, where the new block should be placed. For this example we create a new module which is called joystick.- start scilab
- start the HART-Toolbox
- run the module_creator function of the HART-Toolbox
hart_module_creator('joystick')Now we have a module 'joystick' in which the new block can be placed.
Interface function
Each scicos block is defined by an interface function. This function is written in scilab-code and defines the scicos-block. For more information take a look at Structure of an interface file.- start scilab
- start the HART-Toolbox
- run the hart_edit_scicosblock function of the HART-Toolbox
hart_edit_scicosblock()
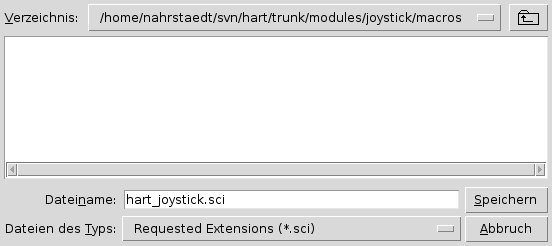
- go to hart/modules/joystick/macros
- choose hart_joystick.sci as file name
- Click on save
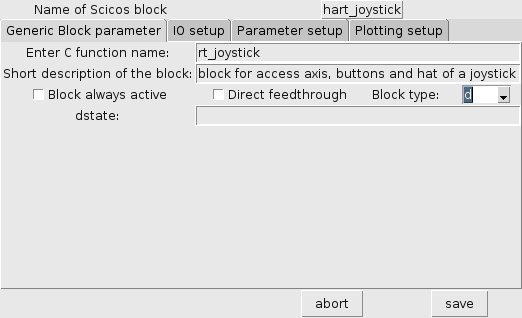
- Enter rt_joystick as C-function name
- choose hart_joystick.sci as file name
- Enter the following for short description: block for access axis, buttons and hat of a joystick
- Click on the next notepad IO setup
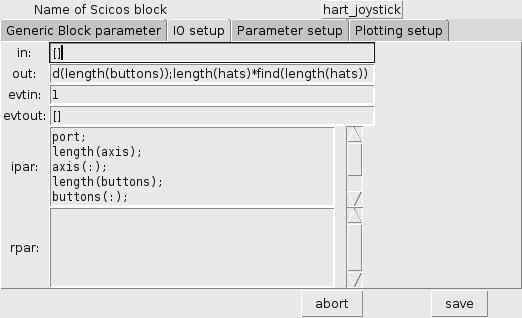
- Leave in free as there should be no input to the block
- The output should be
- There is one red event input: set evntin to 1
- No event output: set evtout to []
- Ipar can be used for sending integer parameters to the C-Function
- There are no floating parameters (rpar)
length(axis)*find(length(axis));length(buttons)*find(length(buttons));length(hats)*find(length(hats))
port;
length(axis);
axis(:);
length(buttons);
buttons(:);
length(hats);
hats(:);
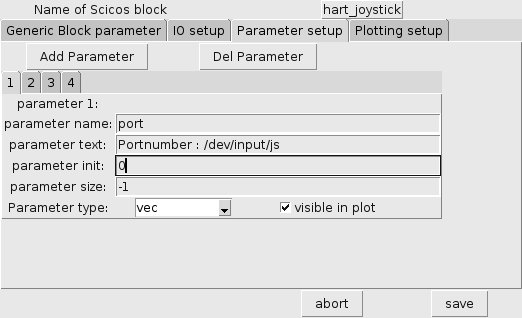
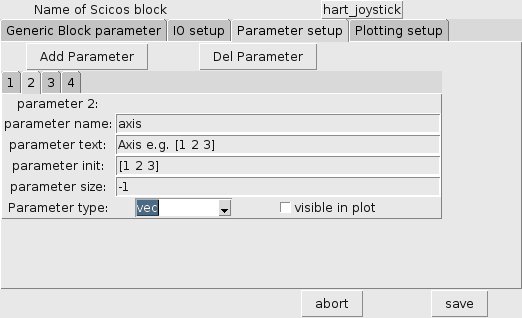
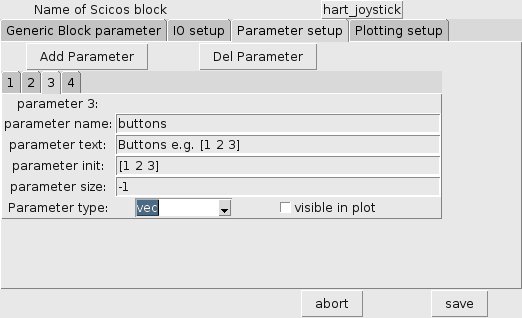
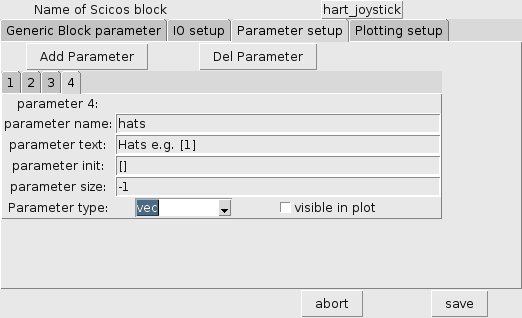
- We need 4 parameters. Click 3 times on add parameter.
- Choose a name, question for the block setup, a initialization and a type.
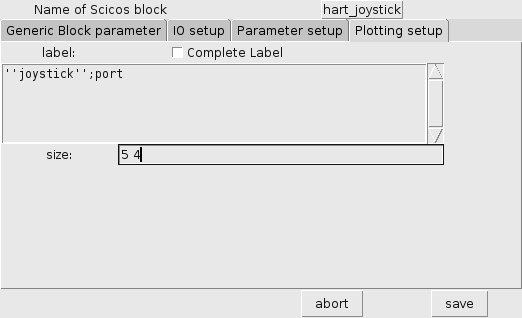
- It is important to use doppel '
- Block will be 5x4 in size.
Computational function
Open a new text file using a text editor of your choice. Save the empty file as hart_joystick.c in joystick/src/c. At fist we need to include some header-files:#include <machine.h>
#include <scicos_block4.h>
#include <SDL.h>
#include <time.h>
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <math.h>
#include <termios.h>
#include <sys/ioctl.h>
#include <signal.h>
#include <pthread.h>
#if defined(RTAI)
#include <rtai_lxrt.h>
#endif
struct oJOY
{
int port;
int Naxis;
int axis;
int Nbutton;
int button;
int Nhat;
int hat;
SDL_Joystick *joystick;
};
static int init(scicos_block *block){
struct oJOY * comdev = (struct oJOY *) malloc(sizeof(struct oJOY));
int i,iparCount=0;
comdev->port = block->ipar[iparCount++];
if ( SDL_Init( SDL_INIT_JOYSTICK) < 0 )
{
fprintf(stderr, "SDL konnte nicht initialisiert werden: %s\n",
SDL_GetError());
}
printf("%i joysticks were found.\n\n", SDL_NumJoysticks() );
printf("The names of the joysticks are:\n");
for( i=0; i < SDL_NumJoysticks(); i++ )
{
printf(" %s\n", SDL_JoystickName(i));
}
comdev->joystick = SDL_JoystickOpen(comdev->port);
comdev->Naxis = block->ipar[iparCount++];
if (comdev->Naxis>0){
comdev->axis = iparCount;
for (i=0;i<comdev->Naxis;i++){
iparCount++;
}
}
if (SDL_JoystickNumAxes(comdev->joystick)<comdev->Naxis)
comdev->Naxis=SDL_JoystickNumAxes(comdev->joystick);
comdev->Nbutton = block->ipar[iparCount++];
if (comdev->Nbutton>0){
comdev->button = iparCount;
for (i=0;i<comdev->Nbutton;i++){
iparCount++;
}
}
if (SDL_JoystickNumButtons(comdev->joystick)<comdev->Nbutton)
comdev->Nbutton=SDL_JoystickNumButtons(comdev->joystick);
comdev->Nhat = block->ipar[iparCount++];
if (comdev->Nhat>0){
comdev->hat = iparCount;
for (i=0;i<comdev->Nhat;i++){
iparCount++;
}
if (SDL_JoystickNumHats(comdev->joystick)<comdev->Nhat)
comdev->Nhat=SDL_JoystickNumHats(comdev->joystick);
}
*block->work=(void *)comdev;
}
static void inout(scicos_block *block){
struct oJOY* comdev = (struct oJOY *) (*block->work);
double *y ;
SDL_JoystickUpdate();
int i,j=0;
int outCounter=1;
//axis
if (comdev->Naxis>0){
y = GetRealOutPortPtrs(block,outCounter);
outCounter++;
for (i=0;i<comdev->Naxis;i++){
y[i]= (double)SDL_JoystickGetAxis(comdev->joystick, block->ipar[comdev->axis+i]-1)/32768.;
}
}
//buttons
if (comdev->Nbutton>0){
y = GetRealOutPortPtrs(block,outCounter);
outCounter++;
for (i=0;i<comdev->Nbutton;i++){
if (SDL_JoystickGetButton(comdev->joystick, block->ipar[comdev->button+i]-1))
y[i]=1;
else
y[i]=0;
}
}
//hat
if (comdev->Nhat>0){
y = GetRealOutPortPtrs(block,outCounter);
outCounter++;
for (i=0;i<comdev->Nhat;i++){
y[i]=(int)SDL_JoystickGetHat(comdev->joystick, block->ipar[comdev->hat+i]-1);
}
}
}
static void end(scicos_block *block){
struct oJOY* comdev = (struct oJOY *) (*block->work);
SDL_JoystickClose(comdev->joystick);
free(comdev);
}
void rt_joystick(scicos_block *block,int flag)
{
if (flag==1){ /* get input */
inout(block);
}
else if (flag==5){ /* termination */
end(block);
}
else if (flag ==4){ /* initialisation */
init(block);
}
}
configure.sce
Change the configure.sce as followingTOOLBOX_NAME = 'input_devices';
TOOLBOX_TITLE = 'input devices module for Hart Toolbox';
if ~MSDOS then
names = ['rt_joystick'];
files = ["hart_joystick.o"];
//check for libsdl.so
sdllib=unix_g('ldconfig -p | grep libSDL');
if (sdllib==[''])
buildable='no';
else
buildable='yes';
ldflags = " -lSDL";
cflags = "-I/usr/include/SDL -D_GNU_SOURCE=1 -D_REENTRANT";
end;
clear sdllib;
else
names = [];
files = [];
disp('sdl-module works only under linux!');
buildable='no';
end